About
Hi there and welcome to my personal site!
My name is Shane R. Sofos. I am an enthusiastic Container Infrastructure Engineer, Application Infrastructure Engineer, Site Reliability Engineer, Systems Engineer, Penguin Wrangler, Pythonist, Rubyist, Rustacean, GNU Herder, Midgardian, Westerosi, Child of Ilúvatar, and have deep experience and passion with automating computer applications and engineering software across the globe in a collaborative way.
I also have an autodidactic experience with the Linux Operating System and thoroughly enjoy using GNU/Linux for a variety of personal projects and hobbies, and in particular compiling different types of software.
The expansive and world changing engineering efforts of the Linux Kernel as the largest globally developed software project has and continues to provide daily inspiration to keep hacking, tinkering, developing, learning, sharing, creating, and having fun.
Code Samples
Identity Class/Struct
# Make some identities
class Identity
attr_accessor :name, :professions
def initialize(give_name, professions)
@name = give_name
@professions = professions
end
def whoami
puts "My name is #{@name}. " +
"I am an enthusiastic #{@professions.join(', ')}."
end
end
'''Small Class to make Identities'''
class Identity:
'''Initialize some identities'''
def __init__(self, given_name, professions):
self.name = given_name
self.professions = professions
def whoami(self):
'''Print an identity'''
professions = ', '.join(self.professions)
print(f'My name is {self.name}. I am an enthusiastic {professions}.')
package Static
type Identity struct {
Name string
Professions string
}
var MyIdentity = []Identity{
{
Name: "Shane R. Sofos",
Professions: "Container Infrastructure Engineer, Application Infrastructure Engineer, Site Reliability Engineer, Systems Engineer, Penguin Wrangler, Pythonist, Rubyist, Rustacean, GNU Herder, Midgardian, Westerosi, Child of Ilúvatar",
},
}
pub struct Whoami {
pub name: &'static str,
pub professions: Vec<&'static str>,
}
pub trait Person {
fn new(name: &'static str, professions: Vec<&'static str>) -> Self;
fn name(&self) -> &'static str;
fn professions(&self) -> String;
}
impl Person for Whoami {
fn new(name: &'static str, professions: Vec<&'static str>) -> Whoami {
Whoami { name, professions }
}
fn name(&self) -> &'static str {
self.name
}
fn professions(&self) -> String {
self.professions.join(", ")
}
}
pub fn about(name: &str, professions: String) -> String {
format!("My name is {}. I am an enthusiastic {}.", name, professions)
}
Identity Instantiation
#!/usr/bin/env ruby
# frozen_string_literal: true
require_relative 'lib/identity'
person = Identity.new(
'Shane R. Sofos',
['Container Infrastructure Engineer',
'Site Reliability Engineer',
'Systems Engineer',
'Penguin Wrangler',
'Pythonist',
'Rubyist',
'Rustacean',
'GNU Herder',
'Midgardian',
'Westerosi',
'and Child of Ilúvatar']
)
person.whoami
#!/usr/bin/env python
'''Print out my identity'''
from identity import Identity
person = Identity(
'Shane R. Sofos',
['Container Infrastructure Engineer',
'Site Reliability Engineer',
'Systems Engineer',
'Penguin Wrangler',
'Pythonist',
'Rubyist',
'Rustacean',
'GNU Herder',
'Midgardian',
'Westerosi',
'and Child of Ilúvatar']
)
person.whoami()
package main
import (
"fmt"
"gitlab.com/ssofos/shanesofos.com/internal/Static"
)
type identity struct {
Name string
Professions string
}
func main() {
for _, id := range Static.MyIdentity {
fmt.Printf("My name is %v. I am an enthusiastic %v.", id.Name, id.Professions)
}
}
use crate::whoami::Person;
mod whoami;
fn main() {
let me: whoami::Whoami = whoami::Person::new(
"Shane R. Sofos",
vec![
"Container Infrastructure Engineer",
"Site Reliability Engineer",
"Systems Engineer",
"Penguin Wrangler",
"Pythonist",
"Rubyist",
"Rustacean",
"GNU Herder",
"Midgardian",
"Westerosi",
"and Child of Ilúvatar",
],
);
println!("{}", whoami::about(me.name, me.professions()));
}
Identity Class Unit Tests
require_relative '../lib/identity'
describe Identity do
before :all do
@person = Identity.new('Tom Bombadil', ['Merry Fellow', 'Master of wood, water and hill'])
end
it 'is an Identity Object' do
expect(@person).to be_kind_of(Identity)
end
it 'responds to 2 arguments' do
expect(Identity).to respond_to(:new).with(2).arguments
end
it 'responds to whoami method' do
expect(@person).to respond_to(:whoami)
end
describe '#name' do
it 'returns a persons name that is a String' do
expect(@person.name).to eq('Tom Bombadil')
expect(@person.name).to be_kind_of(String)
end
end
describe '#professions' do
it 'returns a list of a persons professions that is an Array' do
expect(@person.professions).to eq(['Merry Fellow', 'Master of wood, water and hill'])
expect(@person.professions).to be_kind_of(Array)
end
end
describe '.whoami' do
it 'outputs the identity of a person' do
expect { @person.whoami }.to output('My name is Tom Bombadil. I am an enthusiastic ' +
"Merry Fellow, Master of wood, water and hill.\n").to_stdout
end
end
end
'''Pytest the Identity Class'''
from identity import Identity
IDENTITY = Identity('Tom Bombadil', ['Merry Fellow', 'Master of wood, water and hill'])
def test_identity_name():
'''Test the Identity Class name attribute'''
assert isinstance(IDENTITY.name, str)
def test_identity_professions():
'''Test the Identity Class professions attribute'''
assert isinstance(IDENTITY.professions, list)
def test_identity_whoami(capsys):
'''Test the Identity Class whoami method'''
IDENTITY.whoami()
identity_captured = capsys.readouterr()
assert identity_captured.out == 'My name is Tom Bombadil. ' \
+ 'I am an enthusiastic Merry Fellow, Master of wood, water and hill.\n'
#[cfg(test)]
mod test {
use super::*;
#[test]
fn test_whoami_new() {
let person: Whoami = Person::new("foobar", vec!["fizz", "buzz"]);
assert_eq!(person.name, "foobar");
assert_eq!(person.professions, vec!["fizz", "buzz"]);
}
#[test]
fn test_about() {
let person: Whoami = Person::new("foobar", vec!["fizz", "buzz"]);
let about = about(person.name, person.professions());
assert_eq!(about, "My name is foobar. I am an enthusiastic fizz, buzz.");
}
}
Experience
For more information about my background and skills check out my resume.
Projects
For more info on past and current projects I am working on check out my Projects page.
Contact
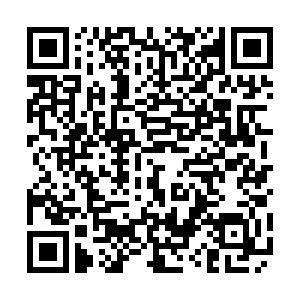